CODECRUSH
Extensible and powerful code editor for Javascript
v0.0.6
StarCore package
If you want to use the editor for your vanilla js without any framework.
Installation
Choose your favorite package manager
_10pnpm install codecrush-core
_10npm install codecrush-core
_10yarn add codecrush-core
Getting started
_15import { initEditor } from "codecrush-core";_15import "codecrush-core/dist/index.css"; // styles from the core package_15_15const app = document.getElementById("app");_15_15if (app) {_15 initEditor({_15 height: 400,_15 id: "js-editor",_15 parent: app,_15 theme: "material-darker",_15 }).then(() => {_15 console.log("editor loaded");_15 });_15}
Themes
List of all themes included
_12export type EditorThemes =_12 | "dracula-soft"_12 | "material-darker"_12 | "material-default"_12 | "material-ocean"_12 | "material-palenight"_12 | "nord"_12 | "one-dark-pro"_12 | "poimandres"_12 | "rose-pine-moon"_12 | "rose-pine"_12 | "slack-dark";
Extending the editor
You can create custom components for the editor. The following example we create a component to register in the activity bar which key is pressed.
In this case we'll be using onReady
and onKeyPressed
events provided by the editor.
_30import { initEditor, Component, ActivityBarComponent } from "codecrush-core";_30import "codecrush-core/dist/index.css"; // styles from the core package_30_30class Example extends Component {_30 onReady() {_30 const activityBar =_30 this.editor.getComponent<ActivityBarComponent>("activity-bar"); // get the activity bar_30 activityBar.registerActivity("key-pressed", "Keyboard: ", false); // register a new entry with id and text_30 }_30_30 onKeyPressed(key: string) {_30 const activityBar =_30 this.editor.getComponent<ActivityBarComponent>("activity-bar");_30 activityBar.updateActivity("key-pressed", "Keyboard: " + key); //update the activity when the key is pressed_30 }_30}_30_30const app = document.getElementById("app");_30_30if (app) {_30 initEditor({_30 height: 400,_30 id: "js-editor",_30 parent: app,_30 theme: "material-darker",_30 components: [Example],_30 }).then(() => {_30 console.log("editor loaded");_30 });_30}
Codecrush React
A wrapper for the core package for react.
Installation
Choose your favorite package manager
_10pnpm install codecrush-core codecrush-react
_10npm install codecrush-core codecrush-react
_10yarn add codecrush-core codecrush-react
Getting started
_12import { Editor } from "codecrush-react";_12import "codecrush-core/dist/index.css"; // styles from the core package_12_12function App() {_12 return (_12 <div className="App">_12 <Editor height={400} id="js-editor" theme="material-darker" />_12 </div>_12 );_12}_12_12export default App;
Themes
List of all themes included
_12export type EditorThemes =_12 | "dracula-soft"_12 | "material-darker"_12 | "material-default"_12 | "material-ocean"_12 | "material-palenight"_12 | "nord"_12 | "one-dark-pro"_12 | "poimandres"_12 | "rose-pine-moon"_12 | "rose-pine"_12 | "slack-dark";
Extending the editor
You can create custom components for the editor. The following example we create a component to register in the activity bar which key is pressed.
In this case we'll be using onReady
and onKeyPressed
events provided by the editor.
_32import { Editor } from "codecrush-react";_32import "codecrush-core/dist/index.css"; // styles from the core package_32import { Component, ActivityBarComponent } from "codecrush-core";_32_32class Example extends Component {_32 onReady() {_32 const activityBar =_32 this.editor.getComponent<ActivityBarComponent>("activity-bar"); // get the activity bar_32 activityBar.registerActivity("key-pressed", "Keyboard: "); // register a new entry with id and text_32 }_32_32 onKeyPressed(key: string) {_32 const activityBar =_32 this.editor.getComponent <ActivityBarComponent>("activity-bar");_32 activityBar.updateActivity("key-pressed", "Keyboard: " + key); //update the activity when the key is pressed_32 }_32}_32_32function App() {_32 return (_32 <div className="App">_32 <Editor_32 height={400}_32 id="js-editor"_32 theme="material-darker"_32 components={[Example]}_32 />_32 </div>_32 );_32}_32_32export default App;
Made by JosueRhea
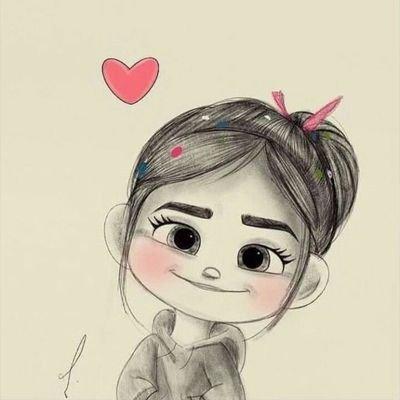